Java練習---學生信息管理程序
思路以及代碼:
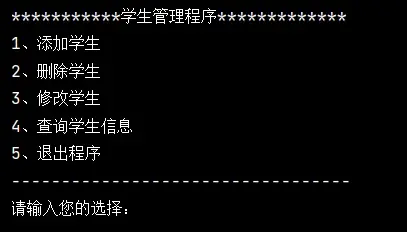
1、首先要創(chuàng)建一個student類,定義student類的屬性例如id、name等。
提供學生類的構造方法和get、set方法。
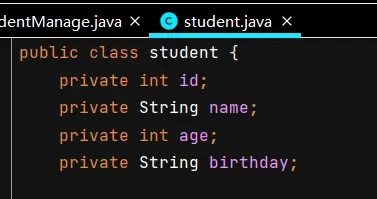
2、new一個集合容器,用于存放student類
3、大概制作一下程序界面,這里使用了一個死循環(huán)。
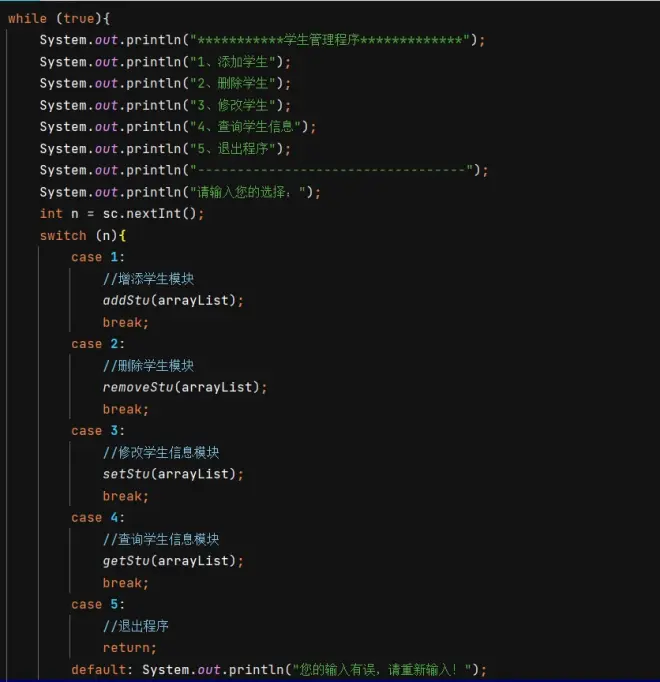
4、增添學生
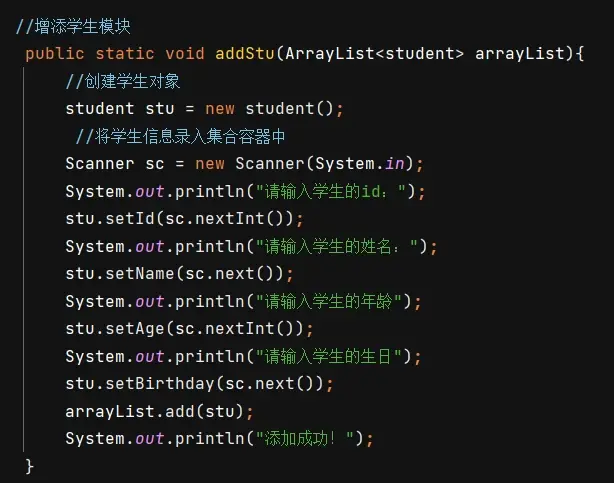
5、查詢學生
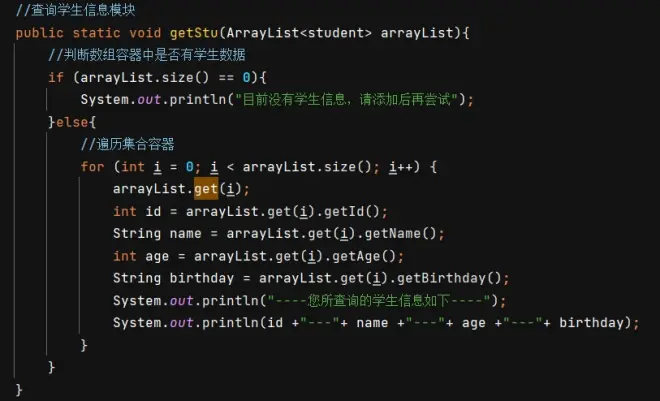
6、修改學生
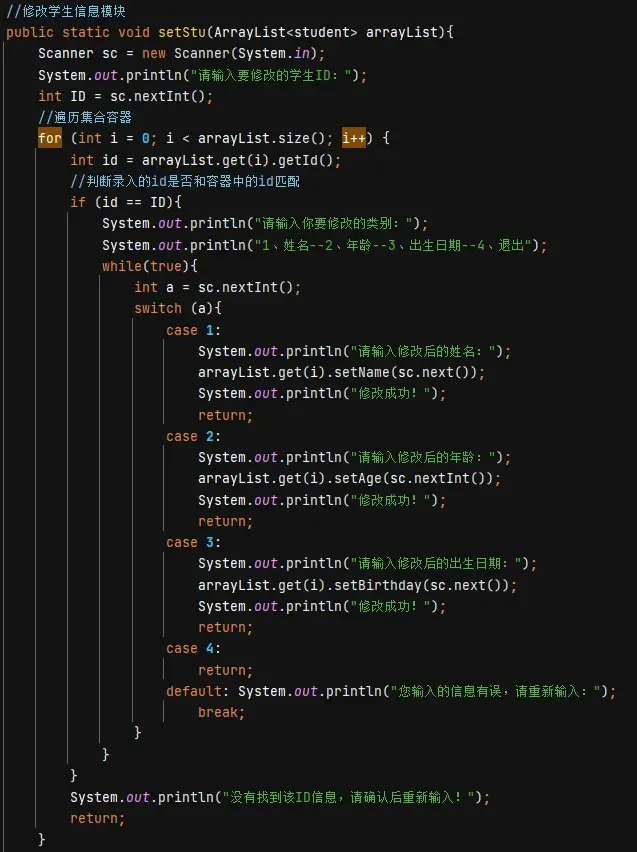
7、刪除學生
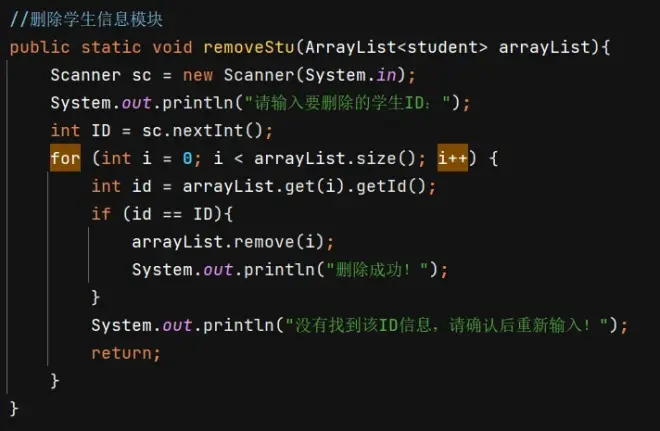
----------------------------------------------------代碼----------------------------------------------------
student類:
public class student {
? ?private int id;
? ?private String name;
? ?private int age;
? ?private String birthday;
? ?public student() {
? ?}
? ?public student(int id, String name, int age, String birthday) {
? ? ? ?this.id = id;
? ? ? ?this.name = name;
? ? ? ?this.age = age;
? ? ? ?this.birthday = birthday;
? ?}
? ?/**
? ? * 獲取
? ? * @return id
? ? */
? ?public int getId() {
? ? ? ?return id;
? ?}
? ?/**
? ? * 設置
? ? * @param id
? ? */
? ?public void setId(int id) {
? ? ? ?this.id = id;
? ?}
? ?/**
? ? * 獲取
? ? * @return name
? ? */
? ?public String getName() {
? ? ? ?return name;
? ?}
? ?/**
? ? * 設置
? ? * @param name
? ? */
? ?public void setName(String name) {
? ? ? ?this.name = name;
? ?}
? ?/**
? ? * 獲取
? ? * @return age
? ? */
? ?public int getAge() {
? ? ? ?return age;
? ?}
? ?/**
? ? * 設置
? ? * @param age
? ? */
? ?public void setAge(int age) {
? ? ? ?this.age = age;
? ?}
? ?/**
? ? * 獲取
? ? * @return birthday
? ? */
? ?public String getBirthday() {
? ? ? ?return birthday;
? ?}
? ?/**
? ? * 設置
? ? * @param birthday
? ? */
? ?public void setBirthday(String birthday) {
? ? ? ?this.birthday = birthday;
? ?}
}
主方法類:
import java.sql.SQLOutput;
import java.util.ArrayList;
import java.util.Scanner;
public class StudentManage {
? ?public static void main(String[] args) {
? ? ? ?Scanner sc = new Scanner(System.in);
? ? ? ?//創(chuàng)建集合容器存儲學生對象
? ? ? ?ArrayList<student> arrayList = new ArrayList<>();
? ? ? ?while (true){
? ? ? ? ? ?System.out.println("***********學生管理程序*************");
? ? ? ? ? ?System.out.println("1、添加學生");
? ? ? ? ? ?System.out.println("2、刪除學生");
? ? ? ? ? ?System.out.println("3、修改學生");
? ? ? ? ? ?System.out.println("4、查詢學生信息");
? ? ? ? ? ?System.out.println("5、退出程序");
? ? ? ? ? ?System.out.println("----------------------------------");
? ? ? ? ? ?System.out.println("請輸入您的選擇:");
? ? ? ? ? ?int n = sc.nextInt();
? ? ? ? ? ?switch (n){
? ? ? ? ? ? ? ?case 1:
? ? ? ? ? ? ? ? ? ?//增添學生模塊
? ? ? ? ? ? ? ? ? ?addStu(arrayList);
? ? ? ? ? ? ? ? ? ?break;
? ? ? ? ? ? ? ?case 2:
? ? ? ? ? ? ? ? ? ?//刪除學生模塊
? ? ? ? ? ? ? ? ? ?removeStu(arrayList);
? ? ? ? ? ? ? ? ? ?break;
? ? ? ? ? ? ? ?case 3:
? ? ? ? ? ? ? ? ? ?//修改學生信息模塊
? ? ? ? ? ? ? ? ? ?setStu(arrayList);
? ? ? ? ? ? ? ? ? ?break;
? ? ? ? ? ? ? ?case 4:
? ? ? ? ? ? ? ? ? ?//查詢學生信息模塊
? ? ? ? ? ? ? ? ? ?getStu(arrayList);
? ? ? ? ? ? ? ? ? ?break;
? ? ? ? ? ? ? ?case 5:
? ? ? ? ? ? ? ? ? ?//退出程序
? ? ? ? ? ? ? ? ? ?return;
? ? ? ? ? ? ? ?default: System.out.println("您的輸入有誤,請重新輸入!");
? ? ? ? ? ?}
? ? ? ?}
? ?}
? //增添學生模塊
? ?public static void addStu(ArrayList<student> arrayList){
? ? ? ?//創(chuàng)建學生對象
? ? ? ?student stu = new student();
? ? ? ? //將學生信息錄入集合容器中
? ? ? ?Scanner sc = new Scanner(System.in);
? ? ? ?System.out.println("請輸入學生的id:");
? ? ? ?stu.setId(sc.nextInt());
? ? ? ?System.out.println("請輸入學生的姓名:");
? ? ? ?stu.setName(sc.next());
? ? ? ?System.out.println("請輸入學生的年齡");
? ? ? ?stu.setAge(sc.nextInt());
? ? ? ?System.out.println("請輸入學生的生日");
? ? ? ?stu.setBirthday(sc.next());
? ? ? ?arrayList.add(stu);
? ? ? ?System.out.println("添加成功!");
? ?}
? ?//查詢學生信息模塊
? ?public static void getStu(ArrayList<student> arrayList){
? ? ? ?//判斷數(shù)組容器中是否有學生數(shù)據(jù)
? ? ? ?if (arrayList.size() == 0){
? ? ? ? ? ?System.out.println("目前沒有學生信息,請?zhí)砑雍笤賴L試");
? ? ? ?}else{
? ? ? ? ? ?//遍歷集合容器
? ? ? ? ? ?for (int i = 0; i < arrayList.size(); i++) {
? ? ? ? ? ? ? ?arrayList.get(i);
? ? ? ? ? ? ? ?int id = arrayList.get(i).getId();
? ? ? ? ? ? ? ?String name = arrayList.get(i).getName();
? ? ? ? ? ? ? ?int age = arrayList.get(i).getAge();
? ? ? ? ? ? ? ?String birthday = arrayList.get(i).getBirthday();
? ? ? ? ? ? ? ?System.out.println("----您所查詢的學生信息如下----");
? ? ? ? ? ? ? ?System.out.println(id +"---"+ name +"---"+ age +"---"+ birthday);
? ? ? ? ? ?}
? ? ? ?}
? ?}
? ?//修改學生信息模塊
? ?public static void setStu(ArrayList<student> arrayList){
? ? ? ?Scanner sc = new Scanner(System.in);
? ? ? ?System.out.println("請輸入要修改的學生ID:");
? ? ? ?int ID = sc.nextInt();
? ? ? ?//遍歷集合容器
? ? ? ?for (int i = 0; i < arrayList.size(); i++) {
? ? ? ? ? ?int id = arrayList.get(i).getId();
? ? ? ? ? ?//判斷錄入的id是否和容器中的id匹配
? ? ? ? ? ?if (id == ID){
? ? ? ? ? ? ? ?System.out.println("請輸入你要修改的類別:");
? ? ? ? ? ? ? ?System.out.println("1、姓名--2、年齡--3、出生日期--4、退出");
? ? ? ? ? ? ? ?while(true){
? ? ? ? ? ? ? ? ? ?int a = sc.nextInt();
? ? ? ? ? ? ? ? ? ?switch (a){
? ? ? ? ? ? ? ? ? ? ? ?case 1:
? ? ? ? ? ? ? ? ? ? ? ? ? ?System.out.println("請輸入修改后的姓名:");
? ? ? ? ? ? ? ? ? ? ? ? ? ?arrayList.get(i).setName(sc.next());
? ? ? ? ? ? ? ? ? ? ? ? ? ?System.out.println("修改成功!");
? ? ? ? ? ? ? ? ? ? ? ? ? ?return;
? ? ? ? ? ? ? ? ? ? ? ?case 2:
? ? ? ? ? ? ? ? ? ? ? ? ? ?System.out.println("請輸入修改后的年齡:");
? ? ? ? ? ? ? ? ? ? ? ? ? ?arrayList.get(i).setAge(sc.nextInt());
? ? ? ? ? ? ? ? ? ? ? ? ? ?System.out.println("修改成功!");
? ? ? ? ? ? ? ? ? ? ? ? ? ?return;
? ? ? ? ? ? ? ? ? ? ? ?case 3:
? ? ? ? ? ? ? ? ? ? ? ? ? ?System.out.println("請輸入修改后的出生日期:");
? ? ? ? ? ? ? ? ? ? ? ? ? ?arrayList.get(i).setBirthday(sc.next());
? ? ? ? ? ? ? ? ? ? ? ? ? ?System.out.println("修改成功!");
? ? ? ? ? ? ? ? ? ? ? ? ? ?return;
? ? ? ? ? ? ? ? ? ? ? ?case 4:
? ? ? ? ? ? ? ? ? ? ? ? ? ?return;
? ? ? ? ? ? ? ? ? ? ? ?default: System.out.println("您輸入的信息有誤,請重新輸入:");
? ? ? ? ? ? ? ? ? ? ? ? ? ?break;
? ? ? ? ? ? ? ? ? ?}
? ? ? ? ? ? ? ?}
? ? ? ? ? ?}
? ? ? ? ? ?System.out.println("沒有找到該ID信息,請確認后重新輸入!");
? ? ? ? ? ?return;
? ? ? ?}
? ?}
? ?//刪除學生信息模塊
? ?public static void removeStu(ArrayList<student> arrayList){
? ? ? ?Scanner sc = new Scanner(System.in);
? ? ? ?System.out.println("請輸入要刪除的學生ID:");
? ? ? ?int ID = sc.nextInt();
? ? ? ?for (int i = 0; i < arrayList.size(); i++) {
? ? ? ? ? ?int id = arrayList.get(i).getId();
? ? ? ? ? ?if (id == ID){
? ? ? ? ? ? ? ?arrayList.remove(i);
? ? ? ? ? ? ? ?System.out.println("刪除成功!");
? ? ? ? ? ?}
? ? ? ? ? ?System.out.println("沒有找到該ID信息,請確認后重新輸入!");
? ? ? ? ? ?return;
? ? ? ?}
? ?}
}